Features¶
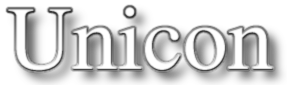
Unicon features¶
Unicon is a feature rich environment. This chapter deals with some of the more miscellaneous components included in Unicon that are not detailed in other sections.
Keyboard functions¶
Unicon supports keyboard scanning outside normal standard IO operation support. This allows for pending key tests, unbuffered input (no Enter key required) and other niceties.
kbhit() checks if there is a keyboard character waiting to be read. getch() reads a key (and will wait if needed) without echo to screen. getche() reads a key (and will wait if needed) with echo to screen.
#
# keyboarding.icn, demonstrate keyboard functions
#
procedure main()
if kbhit() then write(ch := getch())
write("keyscan: ", image(ch))
end
Sample run:
prompt$ unicon -s keyboarding.icn -x
keyscan: &null
Pseudo terminals¶
Qutaiba Mahmoud added support for pseudo terminals in Unicon when attaining his Master’s Degree in Computer Science, outlined in a report hosted along with the Unicon Technical Reports, at
http://www2.cs.uidaho.edu/~jeffery/unicon/reports/mahmoud.pdf
Basically, a file stream is opened with mode "prw"
, which gives a
Unicon master terminal program asynchronous read and write access to another
interactive process.
This feature is similar to the capabilities provided in the famous Expect Tcl/Tk extension by Don Libes.
Todo
add pty sample
libz compression¶
Compression can be used in Unicon file operations, and is also supported in
the compiler for compressed icode generation, if libz
is available
during Unicon build.
unicon -Z program.icn will produce a compressed icode
file. It
will be automatically uncompressed at runtime, assuming support is included
in the current VM when invoked.
Programmers can also use this feature with the "z"
mode modifier of
the open function. Compressed data is not line oriented, so use reads and
writes.
#
# gzio.icn, Demonstrate libz compression
#
link printf
procedure main()
if not find("libz", &features) then stop("no libz compression")
# compress some text, libz adds a little overhead
filename := "gzio.txt.gz"
f := open(filename, "wz") | stop("cannot write ", filename)
writes(f, "First line\n")
every 1 to 4 do writes(f, &ucase || &lcase || "\n")
writes(f, "Last line\n")
close(f)
# image of compressed file
f := open(filename, "r")
data := reads(f, -1)
close(f)
write("Compressed data, size=", *data)
hexdump(data)
# read and uncompress the data
write("\nUncompressed data")
f := open(filename, "rz")
while writes(reads(f))
close(f)
end
#
# display hex codes
#
procedure hexdump(s)
local c, perline := 0
every c := !s do {
if (perline +:= 1) > 16 then write() & perline := 1
writes(map(_doprnt("%02x ", [ord(c)]), &lcase, &ucase))
}
write()
end
Sample run:
prompt$ unicon -s gzio.icn -x
Compressed data, size=89
1F 8B 08 00 00 00 00 00 00 03 73 CB 2C 2A 2E 51
C8 C9 CC 4B E5 72 74 72 76 71 75 73 F7 F0 F4 F2
F6 F1 F5 F3 0F 08 0C 0A 0E 09 0D 0B 8F 88 8C 4A
4C 4A 4E 49 4D 4B CF C8 CC CA CE C9 CD CB 2F 28
04 EA 29 2D 2B AF A8 AC 1A EC 9A 7C 12 61 BE 03
00 22 21 2D 35 E9 00 00 00
Uncompressed data
First line
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz
Last line
Index | Previous: Threading | Next: Documentation