Keywords¶
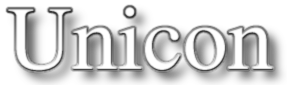
Keywords in Icon and Unicon
provide access to wide
assortment of environmental data, constants, and feature settings and control.
All keywords start with an ampersand symbol &
.
History sidetrip: Unicon keywords are an evolution of SNOBOL
keywords. In SNOBOL
, some keywords were also called trapped
variables
, as accessing the keywords would cause special processing in
SNOBOL
.
Unicon Keywords¶
&allocated¶
- Read-only
- Generates integers
A generator that produces 4 integers; the cumulative number of bytes used for the
- heap
- static
- string
- block
memory regions.
#
# show initial allocations, create a string, reshow allocations
#
procedure main()
allocated()
s := repl(&letters, 1000)
write("\nAfter string creation")
allocated()
end
# Display current memory region allocations
procedure allocated()
local allocs
allocs := [] ; every put(allocs, &allocated)
write("&allocated elements ", *allocs)
write("---------------------")
write("Heap : ", allocs[1])
write("Static : ", allocs[2])
write("String : ", allocs[3])
write("Block : ", allocs[4])
end
Giving:
prompt$ unicon -s allocated.icn -x
&allocated elements 4
---------------------
Heap : 34528
Static : 0
String : 0
Block : 34528
After string creation
&allocated elements 4
---------------------
Heap : 86860
Static : 0
String : 52052
Block : 34808
See also
&ascii¶
#
# Display some of the &ascii keyword, not all, due to unprintables
#
procedure main()
write("Size of &ascii: ", *&ascii)
write("32 bytes starting at offset 65: ", &ascii[65:97])
end
Giving:
prompt$ unicon -s ascii.icn -x
Size of &ascii: 128
32 bytes starting at offset 65: @ABCDEFGHIJKLMNOPQRSTUVWXYZ[\]^_
Note that Icon indexing is 1 relative, so the 65th item is byte code 64.
A handy Cset keyword, but be wary with displaying this value in full, as it includes unprintable control codes.
&clock¶
- Read-only
- Produces a string with the current time in
hh:mm:ss
form.
#
# &clock sample
#
procedure main()
write("&clock: ", &clock)
end
Giving:
prompt$ unicon -s clock.icn -x
&clock: 13:22:37
&col¶
- Read-write
- Produces: an integer
&col
is the mouse horizontal position in text columns, from the most
recent Event(). If &col
is assigned, &x is set to a
corresponding pixel location using the current font of &window.
#
# col.icn, demonstrate the &col mouse column keyword
#
link enqueue, evmux
procedure main()
window := open("mouse column", "g", "size=20,20", "canvas=hidden")
Enqueue(window, &lpress, 11, 14, "", 2)
w := Active()
write(image(w))
e := Event(w, 1)
write("event at mouse column ", &col)
write("event at mouse position (", &x, ",", &y, ")")
close(window)
end
Sample run:
prompt$ unicon -s col.icn -x
window_1:1(mouse column)
event at mouse column 2
event at mouse position (11,14)
&collections¶
- Read-only
- Generates integers
A generator that produces 4 integers; the number of times memory has been reclaimed from the
- heap
- static
- string
- block
memory regions.
#
# show collections, create and remove a string, reshow collections
#
procedure main()
collections()
s := repl(&letters, 1000)
s := &null
collect()
write("\nAfter string create/remove")
collections()
end
# Display current memory region allocations
procedure collections()
local collects
collects := [] ; every put(collects, &collections)
write("Collections, ", *collects, " values generated")
write(repl("-", 30 + **collects))
write("Heap : ", collects[1])
write("Static : ", collects[2])
write("String : ", collects[3])
write("Block : ", collects[4])
end
Giving:
prompt$ unicon -s collections.icn -x
Collections, 4 values generated
-------------------------------
Heap : 0
Static : 0
String : 0
Block : 0
After string create/remove
Collections, 4 values generated
-------------------------------
Heap : 1
Static : 0
String : 0
Block : 0
See also
&column¶
- Read-only
- Returns an integer
Produces the integer column, from the program source code, of the current execution point.
#
# Display the current source column
#
procedure main()
write("Executing code from column: ", &column)
end
Giving:
prompt$ unicon -s column.icn -x
Executing code from column: 10
&control¶
- Read-only
- Produces: &null or failure
null if control key was down on last X event, otherwise a reference to &control fails.
#
# control.icn, demonstrate the &control key status keyword
#
link enqueue, evmux
procedure main()
window := open("control", "g", "size=20,20", "canvas=hidden")
# Enqueue an event with a "c" modifier, setting the control key state
Enqueue(window, &lpress, 11, 14, "c", 2)
Enqueue(window, &lrelease, 11, 14, "", 2)
w := Active()
write(image(w))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
write("&control: ", image(&control))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
write("&control: ", image(&control))
close(window)
end
Sample run:
prompt$ unicon -s control.icn -x
window_1:1(control)
event at mouse position (11,14)
&control: &null
event at mouse position (11,14)
&cset¶
- Read-only
- Produces a cset that includes all characters.
#
# Display some of the &cset keyword, not all, due to unprintables
#
procedure main()
write("Size of &cset: ", *&cset)
write("32 bytes starting at offset 41: ", &cset[41:73])
end
Giving:
prompt$ unicon -s cset.icn -x
Size of &cset: 256
32 bytes starting at offset 41: ()*+,-./0123456789:;<=>?@ABCDEFG
Note that Icon indexing is 1 relative, so the 41st item is byte code 40.
A very handy keyword, as subsets of character sequences can easily
be made by indexing &cset
. Includes unprintable character codes.
¤t¶
- Read-only
- Produces a co-expression.
Produces the co-expression that is currently executing.
#
# ¤t sample, using image for this unprintable datatype
#
procedure main()
# create a co-expression
coex := create {
write("\t¤t in coex: ", image(¤t))
write("\t&source in coex: ", image(&source))
}
# show ¤t in main
if ¤t === &source then
write("¤t in main: ", image(¤t))
else
write("problem: ¤t not equal &source")
# now show ¤t in the co-expression
@coex
end
Giving:
prompt$ unicon -s current.icn -x
¤t in main: co-expression_1(1)
¤t in coex: co-expression_2(0)
&source in coex: co-expression_1(1)
See also
Side comment: co-expressions, are cool.
&date¶
- Read-only
- Produces a string with the current date in
yyyy/mm/dd
form.
#
# &date sample
#
procedure main()
write("&date: ", &date)
# transpose to month/day/year form
write("M/D/Y: ", map("Mm/Dd/yYxX", "yYxX/Mm/Dd", &date))
end
Giving:
prompt$ unicon -s date.icn -x
&date: 2019/10/25
M/D/Y: 10/25/2019
The sample code highlights a powerful Unicon idiom, for transposing strings
around, called labelling
. It converts from system yyyy/mm/dd
to
mm/dd/yyyy
format.
If you prefer other forms, the advanced transposition features of function map can come in handy.
map("Dd/Mm/yYxX", "yYxX/Mm/Dd", &date)
Will map the date from yyyy/mm/dd to dd/mm/yyyy form. Note this map is in (transpositions, labels, source) order. The second argument (the labels) can have no duplicate characters, and must be the same size as the third argument for, the transposition type mapping to occur.
But, stick with the default &date
format if you can. It’s not
ambiguous, and it’s easily machine sortable.
&dateline¶
- Read-only
- Produces a string with the current date in human readable form.
The &dateline
keyword gives a nice time stamp, with day of the week, date
and time (to the minute).
#
# &dateline sample
#
procedure main()
write("&dateline: ", &dateline)
end
Giving:
prompt$ unicon -s dateline.icn -x
&dateline: Friday, October 25, 2019 1:22 pm
&digits¶
- Read-only
- Produces a cset that includes the ten decimal digits.
#
# Display the &digits keyword cset
#
procedure main()
write("Size of &digits: ", *&digits)
write("Cset of &digtis: ", &digits)
end
Giving:
prompt$ unicon -s digits.icn -x
Size of &digits: 10
Cset of &digtis: 0123456789
&dump¶
- Read-Write
- An integer that controls the generation of a program dump.
If &dump
is non-zero when a program halts, all local and global variables
are shown, along with their values.
#
# Display an end of run storage dump
#
procedure main()
write("Dump variables on exit")
a := 1
b := 1.0
c := &digits
d := "Unicon"
e := &letters || &digits
f := write
g := [1,2,3,4]
&dump := 1
stop("&dump is ", &dump)
end
Sample run (ends with a stop, error code set to 1, the value of &dump
):
prompt$ unicon -s dump.icn -x
Dump variables on exit
&dump is 1
Termination dump:
co-expression #1(1)
main local identifiers:
a = 1
b = 1.0
c = &digits
d = "Unicon"
e = "ABCDEFGHIJKLMNOP..."
f = function write
g = list_1 = [1,2,3,4]
global identifiers:
main = procedure main
stop = function stop
write = function write
&e¶
- Read-only
- Produces a Real, representing Euler’s number, the base of the natural logarithms.
e
is a marvelous, magical number.
#
# &e, Euler's number, the natural logarithm
#
procedure main()
write("Size of &e: ", *&e)
write("Value of &e: ", &e)
end
Giving:
prompt$ unicon -s e.icn -x
Size of &e: 17
Value of &e: 2.718281828459045
&errno¶
- Read-only
- Produces: integer
Variable containing transient error number from previous POSIX command.
#
# errno.icn, demonstrate the POSIX volatile &errno value
#
procedure main()
# attempt POSIX operation on non existent file
write("attempt readlink on non-existent-file")
readlink("non-existent-file")
write("&errno: ", &errno)
write("errno 2 is ENOENT (No such file or directory) in Linux")
end
Sample run:
prompt$ unicon -s errno.icn -x
attempt readlink on non-existent-file
&errno: 2
errno 2 is ENOENT (No such file or directory) in Linux
&error¶
- Read-Write
- An integer that controls how runtime errors are handled.
If &error
is non-zero then runtime errors are converted into expression
failure. &error
is decremented each time a runtime error occurs. Setting
&error
to -1 (minus one) effectively disables runtime errors indefinitely.
#
# Runtime error conversion to failure with &error
#
procedure main()
&error := 2
every i := 1 to 3 do {
write("attempt: ", i, " &error is ", &error)
a := "1" + []
}
end
Sample run (eventually ends with failure):
prompt$ unicon -s error.icn -x
attempt: 1 &error is 2
attempt: 2 &error is 1
attempt: 3 &error is 0
Run-time error 102
File error.icn; Line 15
numeric expected
offending value: list_3 = []
Traceback:
main()
{1 + list_3 = []} from line 15 in error.icn
Side comment: Unicon style expression failure, should be in every language.
See also
&errornumber¶
- Read-only
- Produces an integer
&errornumber
is the number of the last error converted to failure, if any.
#
# Runtime error conversion to failure; &errornumber
#
procedure main()
&error := 2
write("&errornumber: ", &errornumber)
every i := 1 to 3 do {
write("attempt: ", i, " &error is ", &error)
a := "1" + []
write("&errornumber: ", &errornumber)
}
end
Sample run (eventually ends with failure code):
prompt$ unicon -s errornumber.icn -x
attempt: 1 &error is 2
&errornumber: 102
attempt: 2 &error is 1
&errornumber: 102
attempt: 3 &error is 0
Run-time error 102
File errornumber.icn; Line 16
numeric expected
offending value: list_3 = []
Traceback:
main()
{1 + list_3 = []} from line 16 in errornumber.icn
On program startup, &errornumber
is undefined, the first write
expression fails.
See also
&errortext¶
- Read-only
- Produces a string
&errortext
is the last error message that was converted to failure, if any.
#
# Runtime error conversion to failure; &errortext
#
procedure main()
&error := 2
write("&errortext: ", &errortext)
every i := 1 to 3 do {
write("attempt: ", i, " &error is ", &error)
a := "1" + []
write("&errortext: ", &errortext)
}
end
Sample run (eventually ends with failure code):
prompt$ unicon -s errortext.icn -x
&errortext:
attempt: 1 &error is 2
&errortext: numeric expected
attempt: 2 &error is 1
&errortext: numeric expected
attempt: 3 &error is 0
Run-time error 102
File errortext.icn; Line 16
numeric expected
offending value: list_3 = []
Traceback:
main()
{1 + list_3 = []} from line 16 in errortext.icn
On program startup, &errortext
is undefined, the first write
expression fails.
See also
&errorvalue¶
- Read-only
- Produces a value
&errorvalue
is the value involved in the last error that was converted to
failure, if any.
#
# Runtime error conversion to failure; &errorvalue
#
procedure main()
&error := 2
write("&errorvalue: ", image(&errorvalue))
every i := 1 to 3 do {
write("attempt: ", i, " &error is ", &error)
a := "1" + []
write("&errorvalue: ", image(&errorvalue))
}
end
Sample run (eventually ends with failure code):
prompt$ unicon -s errorvalue.icn -x
attempt: 1 &error is 2
&errorvalue: list_1(0)
attempt: 2 &error is 1
&errorvalue: list_2(0)
attempt: 3 &error is 0
Run-time error 102
File errorvalue.icn; Line 16
numeric expected
offending value: list_3 = []
Traceback:
main()
{1 + list_3 = []} from line 16 in errorvalue.icn
On program startup, &errorvalue
is undefined. As this value can be any
value, image
is used to show the type of value.
See also
&errout¶
- Read-only
- Produces the current standard error file stream
#
# &errout, the standard error stream
#
procedure main()
write(type(&errout))
write(image(&errout))
end
Giving:
prompt$ unicon -s errout.icn -x
file
&errout
&eventcode¶
- Read-only
- Produces: An integer
Event code in monitored program, set from last EvGet
#
# eventcode.icn, demonstrate Execution Monitoring eventcode
# needs 1to4.icn as the monitoring target
#
link evinit, wrap
procedure main()
wrap()
EvInit("1to4")
while EvGet() do write(wrap(left(ord(&eventcode) || ", ",5), 64))
write(wrap())
end
Sample run:
prompt$ unicon -s eventcode.icn -x
189, 185, 160, 160, 186, 67, 188, 187, 160, 160, 162, 161,
188, 187, 160, 161, 160, 160, 160, 191, 160, 160, 191, 160,
160, 191, 160, 187, 160, 176, 189, 185, 179, 187, 160, 176,
73, 75, 78, 73, 75, 115, 115, 177, 187, 160, 186, 243,
1
99, 170, 73, 75, 78, 173, 160, 160, 169, 180, 190, 185,
189, 185, 179, 187, 160, 176, 73, 75, 78, 73, 75, 115,
2
115, 177, 187, 160, 186, 243, 99, 170, 73, 75, 78, 173,
160, 160, 169, 180, 190, 185, 189, 185, 179, 187, 160, 176,
73, 75, 78, 73, 75, 115, 115, 177, 187, 160, 186, 243,
3
99, 170, 73, 75, 78, 173, 160, 160, 169, 180, 190, 185,
189, 185, 179, 187, 160, 176, 73, 75, 78, 73, 75, 115,
4
115, 177, 187, 160, 186, 243, 99, 170, 73, 75, 78, 173,
160, 160, 169, 180, 190, 185, 178, 169, 187, 160, 188, 187,
160, 166, 160, 88,
See also
&eventsource¶
- Read-only
- Produces: A co-expression
Source co-expression of event in monitoring program, set during EvGet.
#
# eventsource.icn, demonstrate Execution Monitoring eventsource
# needs 1to4.icn as the monitoring target
#
link evinit
procedure main()
EvInit("1to4")
EvGet()
write(image(&eventsource))
while EvGet()
end
Sample run:
prompt$ unicon -s eventsource.icn -x
co-expression_1(0)
1
2
3
4
See also
&eventvalue¶
- Read-only
- Produces: any
Value being processed when EvGet returns an execution monitoring event.
#
# eventvalue.icn, demonstrate Execution Monitoring eventvalue
# needs 1to4.icn as the monitoring target
#
link evinit, wrap
procedure main()
wrap()
EvInit("1to4")
while EvGet() do write(wrap(image(&eventvalue) || ", ", 64))
write(wrap())
end
Sample run:
prompt$ unicon -s eventvalue.icn -x
0, 17576205559321, 98, 61, 17576205559321, procedure main, 11,
23068683, 98, 67, 9, 140609644474276, 12, 11075596, 85, 0, 84,
69, 77, "\n", 69, 60, 1, 98, 60, 4, 73, 58720268, 45,
function ..., 5, 17576205559350, 1, 46137356, 3, function ||,
"\n", "", "\n", 1, "", 1, 2, "\n1", 33554444, 61,
1
17576205559358, "write+", 61, function write, "\n1", "", "\n1",
"\n1", 70, 53, -1, 0, 1, 17576205559350, 5, 17576205559350, 2,
46137356, 3, function ||, "\n", "", "\n", 2, "", 1, 2, "\n2",
33554444, 61, 17576205559358, "write+", 61, function write,
2
"\n2", "", "\n2", "\n2", 70, 53, -1, 0, 1, 17576205559350, 5,
17576205559350, 3, 46137356, 3, function ||, "\n", "", "\n", 3,
"", 1, 2, "\n3", 33554444, 61, 17576205559358, "write+", 61,
3
function write, "\n3", "", "\n3", "\n3", 70, 53, -1, 0, 1,
17576205559350, 5, 17576205559350, 4, 46137356, 3, function ||,
"\n", "", "\n", 4, "", 1, 2, "\n4", 33554444, 61,
4
17576205559358, "write+", 61, function write, "\n4", "", "\n4",
"\n4", 70, 53, -1, 0, 1, 17576205559350, function ..., -1,
11141132, 69, 13, 2097165, 68, procedure main, 48, 0,
See also
&fail¶
- Read-only, but fails when accessed
- Causes failure, as soon as it is evaluated.
#
# &fail, immediate expression failure when evaluated
#
procedure main()
write(type(&fail))
write(image(&fail))
end
Giving:
prompt$ unicon -s fail-keyword.icn -x
Which produces no output; both of the expressions, type
and image
fail,
so write
is never evaluated.
See also
&features¶
- Read-only
- Generates string data that indicates the optional, and
non-portable features supported by the current
unicon
build.
A reflective aspect of Unicon
that can be used for purely informational
purposes, or to decide at runtime what code fragments are safe to use on the
current platform.
#
# features.icn, Display the optional and non-portable feature set
#
procedure main()
every write(&features)
if &features == "POSIX" then
write("\nPOSIX code supported with this ", &version)
f := 0
every function() do f +:= 1
write(f, " built in functions")
end
Giving:
prompt$ unicon -s features.icn -x
UNIX
POSIX
DBM
ASCII
co-expressions
native coswitch
concurrent threads
dynamic loading
environment variables
event monitoring
external functions
keyboard functions
large integers
multiple programs
pattern type
pipes
pseudo terminals
system function
messaging
graphics
3D graphics
X Windows
libz file compression
JPEG images
PNG images
SQL via ODBC
Audio
secure sockets layer encryption
CCompiler gcc 5.5.0
Physical memory: 7808401408 bytes
Revision 6034-743ffd1
Arch x86_64
CPU cores 4
Binaries at /home/btiffin/unicon-git/bin/
POSIX code supported with this Unicon Version 13.1. August 19, 2019
302 built in functions
The unicon -feature
command line option will display the &features
data as well.
See also
&file¶
- Read-only
- Produces the string of the source used to compile the current execution point.
#
# &file, Current source file
#
procedure main()
write(&file)
end
Giving:
prompt$ unicon -s file.icn -x
file.icn
&host¶
- Read-only
- Produces a string representing the current network hostname.
#
# &host, the network host name
#
procedure main()
write(&host)
end
Giving:
prompt$ unicon -s host.icn -x
btiffin-CM1745
See also
&input¶
- Read-only
- Produces a file representing the standard input stream.
#
# &input, the standard input stream
#
procedure main()
write(type(&input))
write(image(&input))
end
Giving:
prompt$ unicon -s input.icn -x
file
&input
&interval¶
- Read-only
- Produces: An integer
Milliseconds since previous windowing event.
#
# interval.icn, demonstrate the &interval event timing
#
link enqueue, evmux
procedure main()
window := open("interval", "g", "size=20,20", "canvas=hidden")
# enqueue a press, interval 2ms
Enqueue(window, &lpress, 11, 14, "", 2)
# enqueue a release, interval 3ms
Enqueue(window, &lrelease, 12, 15, "", 3)
w := Active()
write(image(w))
e := Event(w, 1)
write("event interval ", &interval, " ms")
e := Event(w, 1)
write("event interval ", &interval, " ms")
close(window)
end
Sample run:
prompt$ unicon -s interval.icn -x
window_1:1(interval)
event interval 2 ms
event interval 3 ms
See also
&lcase¶
- Read-only
- Produces a cset of the lower case letters, a through z.
#
# &lcase keyword, lower case letters Cset
#
procedure main()
write("Size of &lcase: ", *&lcase)
write(&lcase)
end
Giving:
prompt$ unicon -s lcase.icn -x
Size of &lcase: 26
abcdefghijklmnopqrstuvwxyz
&ldrag¶
- Read-only
- Produces: the integer that indicates a left mouse button drag event code.
left button drag event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&letters¶
- Read-only
- Produces a cset of all letter, A-Za-z.
#
# &letters keyword, upper and lower case letters Cset
#
procedure main()
write("Size of &letters: ", *&letters)
write(&letters)
end
Giving:
prompt$ unicon -s letters.icn -x
Size of &letters: 52
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz
&level¶
- Read-only
- Produces the current execution depth, one level for each nested procedure.
#
# &level, procedure execution depth
#
procedure main()
write("main level: ", &level)
subproc()
end
procedure subproc()
write("subp level: ", &level)
end
Giving:
prompt$ unicon -s level.icn -x
main level: 1
subp level: 2
&line¶
- Read-only
- Produces an integer.
Returns the integer line, from the program source code, of the current execution point.
#
# Display the current source line
#
procedure main()
write("Executing code from line: ", &line)
end
Giving:
prompt$ unicon -s line.icn -x
Executing code from line: 12
&lpress¶
- Read-only
- Produces: the integer that indicates a left mouse button press event code.
left button press event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&lrelease¶
- Read-only
- Produces: the integer that indicates a left mouse button release event code.
left button release event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&main¶
- Read-only
- Produces the main co-expression
#
# &main, main co-expression
#
procedure main()
if ¤t === &main then write("¤t is &main")
coex := create(if &source === &main then write("coex: &source is &main"))
@coex
end
Giving:
prompt$ unicon -s main.icn -x
¤t is &main
coex: &source is &main
&mdrag¶
- Read-only
- Produces: the integer that indicates a middle mouse button drag event code.
middle button drag event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&meta¶
- Read-only
- Produces: &null or fail
null if meta key was down on last X event, otherwise a reference to &meta
fails.
#
# meta.icn, demonstrate the &meta key status keyword
#
link enqueue, evmux
procedure main()
window := open("meta", "g", "size=20,20", "canvas=hidden")
# Enqueue an event with the "m" modifier, setting the meta key state
Enqueue(window, &lpress, 11, 14, "m", 2)
Enqueue(window, &lrelease, 11, 14, "", 2)
w := Active()
write(image(w))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
write("&meta: ", image(&meta))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
write("&meta: ", image(&meta))
close(window)
end
Sample run:
prompt$ unicon -s meta.icn -x
window_1:1(meta)
event at mouse position (11,14)
&meta: &null
event at mouse position (11,14)
&mpress¶
- Read-only
- Produces: the integer that indicates a middle mouse button press event code.
middle button press event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&mrelease¶
- Read-only
- Produces: the integer that indicates a middle mouse button release event code.
middle button release event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&now¶
- Read-only
- Produces an integer representing the current time as a count of seconds since the Unix epoch reference standard, which is midnight January 1st, 1970.
#
# &now sample
#
procedure main()
write("&now :", &now)
delay(1000)
write("and &now :", &now, ", 1000 milliseconds(ish) later")
end
Giving:
prompt$ unicon -s now.icn -x
&now :1572024161
and &now :1572024162, 1000 milliseconds(ish) later
&null¶
- Read-only
- Represents the null value.
The null value is a special case in Unicon
. Unset variables
return &null, and unused arguments test as &null. There are some operators
that help manage this special value.
\var
will fail if the variablevar
is null/var
will succeed and return the (empty) variable reference ifvar
is null which is great for setting optional default values
#
# &null, the null value
#
# b will be given a default value, a will remain unset
procedure main()
\a := "a"
/b := "b"
if a === &null then write("a is &null")
if b === &null then write("b is &null") else write("b is ", image(b))
end
Giving:
prompt$ unicon -s null.icn -x
a is &null
b is "b"
&output¶
- Read-only
- Produces the standard output stream
#
# &output, the standard output stream
#
procedure main()
write(type(&output))
write(image(&output))
end
Giving:
prompt$ unicon -s output.icn -x
file
&output
&phi¶
- Read-only
- Produces a real constant equal to the Golden Ratio
#
# &phi, the constant representing the Golden ratio
#
procedure main()
write(&phi)
end
Giving:
prompt$ unicon -s phi.icn -x
1.618033988749895
&pi¶
- Read-only
- Produces a Real representing the ratio of the diameter of a circle compared to the radius.
#
# &pi, the ratio of the diameter of a circle compared to the radius
#
procedure main()
write(&pi)
write("Size of &pi in string form: ", *&pi)
end
Giving:
prompt$ unicon -s pi.icn -x
3.141592653589793
Size of &pi in string form: 17
&pick¶
- Read-only
- Generates: string* [3D Graphics]
pick
generates the object IDs selected at point(&x, &y) from
the most recent windowing Event; if the event was read from a 3D
window with attribute “pick=on”. Objects need to be registered with
WSection so Unicon knows which elements to generate for &pick
.
#
# pick.icn, demonstrate 3D pick object ID during windowing event
#
link enqueue, evmux
procedure main()
window := open("pick", "gl", "size=90,60")
WAttrib(window, "pick=on")
# mark a named 3D object
WSection(window, "sphere")
DrawSphere(window, 0.0, 0.19, -2.2, 0.3)
Refresh(window)
WSection(window)
# insert an event into the queue, left press, 2ms interval, on sphere
#Enqueue(window, &lpress, 45,20, "", 2)
e := Event(window)
write(image(e))
# a side effect of the Event function is keywords settings
write("&x:", &x)
write("&y:", &y)
write("&row:", &row)
write("&col:", &col)
every write("&pick:", &pick)
WriteImage(window, "../images/pick.png")
close(window)
end
Sample run, clicking on the sphere:
prompt$ unicon -s pick.icn -x
-1
&x:46
&y:24
&row:2
&col:8
&pick:sphere

&pos¶
- Read-write
- Holds the current string scanning position, as an integer.
&pos is set to 1 at the start of a string scanning expression. &pos can be set to arbitrary values, but will be constrained internally to always be a valid string scanning index. When set to a negative indexing value, it will be reset to a valid positive index value.
#
# &pos, string scanning position
#
# demonstrate how negative position indexes are set to actual
#
procedure main()
str := &letters
str ? {
first := &pos
&pos := 0
last := &pos
&pos := -10
back10 := &pos
}
write("first: ", first, ", last: ", last, ", -10: ", back10)
end
Giving:
prompt$ unicon -s pos.icn -x
first: 1, last: 53, -10: 43
See also
&progname¶
- Read-only
- Returns a string
Produces the currently executing program name.
#
# &progname, Current program name
#
procedure main()
write(&progname)
end
Giving:
prompt$ unicon -s progname-sample.icn -x
progname-sample
&random¶
- Read-write
- Produces an integer from the internal pseudo-random number generator used by the unary ? operator.
The &random
seed is initialized to a different value by Unicon for each
program run, but can be set to a known value for reproducible sequences during
testing.
#
# &random seed, seeds the internal pseudo-random sequencer used by unary ?
#
procedure main()
write(&random)
every 1 to 3 do writes(?&letters)
write()
write()
&random := 1
every 1 to 3 do writes(?&letters)
write()
write(&random)
end
Giving:
prompt$ unicon -s random.icn -x
20203448
Yxr
lwQ
686405327
The first value and set of letters will be random (psuedo-random) for every
run, the seed uniquely initialized at program startup. The second set of
characters and the last numeric value will be the same for every run, using a
known seed value of 1
.
When testing you can set a known seed to get a predictable, and consistent sequence of random values. Otherwise just let Unicon reset the seed for each run, and the results are almost[1] unpredictable. Don’t set a known value for things like games that need random elements, or each play through will be predictable and in the worst case, the same, over and over again.
prompt$ unicon -quiet -s random.icn -x
20203463
Jnu
lwQ
686405327
[1] | Psuedo-random number generators are never completely unpredictable, given enough effort. If you need cryptographically secure random values, you will need to augment the default algorithms used in Unicon and mix things up a bit, in order to defeat any bad actors that may try and predict the order and numeric sequences of the psuedo-random numbers. Even players out to cheat on your game might try and guess at sequencing, so mix it up. |
&rdrag¶
- Read-only
- Produces: the Integer that indicates a right mouse button drag event
right button drag.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
®ions¶
- Read-only
- Generates 3 Integers
A generator that produces 3 Integers; the cumulative number of bytes used for the
- static (always zero in Unicon, for backward compatibility)
- string
- block
memory regions.
#
# ®ions, current static, string and block memory sizes
#
procedure main()
region := [] ; every put(region, ®ions)
write("Regions ", *region)
write("---------")
write("Static : ", region[1])
write("String : ", region[2])
write("Block : ", region[3])
end
Giving:
prompt$ unicon -s regions.icn -x
Regions 3
---------
Static : 0
String : 140007669
Block : 140007669
See also
&resize¶
- Read-only
- Produces: Integer
Windowing resize event code.
#
# resize.icn, display the code representing a resize event
#
procedure main()
write("&resize: ", &resize)
end
Sample run:
prompt$ unicon -s resize.icn -x
&resize: -10
See also
&row¶
- Read-only
- Produces: Integer for the effective row of a windowing event
mouse vertical position in text rows.
#
# row.icn, demonstrate &row event keyword
#
link enqueue, evmux
procedure main()
window := open("Event", "g", "size=20,20", "canvas=hidden")
# insert an event into the queue, left press, control/shift, 2ms
Enqueue(window, &lpress, 11, 14, "m", 2)
e := Event(window)
write(image(e))
# a side effect of the Event function is keywords settings
write("&x:", &x)
write("&y:", &y)
write("&row:", &row)
write("&col:", &col)
write("&interval:", &interval)
write("&control:", &control)
write("&shift:", &shift)
write("&meta:", &meta)
close(window)
end
Sample run:
prompt$ unicon -s row.icn -x
-1
&x:11
&y:14
&row:2
&col:2
&interval:2
&meta:
&rpress¶
- Read-only
- Produces: the Integer that indicates a right mouse button press event
Right mouse button press event code.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&rrelease¶
- Read-only
- Produces: the Integer that indicates a right mouse button release event code.
right button release.
#
# button-values.icn, display the left, middle and right mouse button
# event codes for press, release and drag
#
procedure main()
write("&lpress: ", &lpress)
write("&mpress: ", &mpress)
write("&rpress: ", &rpress)
write("&lrelease: ", &lrelease)
write("&mrelease: ", &mrelease)
write("&rrelease: ", &rrelease)
write("&ldrag: ", &ldrag)
write("&mdrag: ", &mdrag)
write("&rdrag: ", &rdrag)
end
Sample run:
prompt$ unicon -s button-values.icn -x
&lpress: -1
&mpress: -2
&rpress: -3
&lrelease: -4
&mrelease: -5
&rrelease: -6
&ldrag: -7
&mdrag: -8
&rdrag: -9
&shift¶
- Read-only
- Produces: &null or fail
null if shift key was down on last X event, otherwise a reference to
&shift
with fail.
#
# shift.icn, demonstrate the &shift key status keyword
#
link enqueue, evmux
procedure main()
window := open("shift", "g", "size=20,20", "canvas=hidden")
# Enqueue an event with the "s" modifier, setting the shift key state
Enqueue(window, &lpress, 11, 14, "s", 2)
Enqueue(window, &lrelease, 11, 14, "", 2)
w := Active()
write(image(w))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
write("&shift: ", image(&shift))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
write("&shift: ", image(&shift))
close(window)
end
Sample run:
prompt$ unicon -s shift.icn -x
window_1:1(shift)
event at mouse position (11,14)
&shift: &null
event at mouse position (11,14)
&source¶
- Read-only
- Produces the source Unicon Co-Expressions of the currently running co-expression.
#
# &source, Source co-expression
#
procedure main()
coex := create(if &source === &main then write("&source is &main"))
@coex
end
Giving:
prompt$ unicon -s source.icn -x
&source is &main
&storage¶
- Read-only
- Generates 3 Integers
A generator that produces 3 Integers; the bytes used for the
- static (always zero in Unicon, for backward compatibility)
- string
- block
memory regions.
#
# &storage, current static, string and block memory usage
#
procedure main()
storage()
str := repl(&ascii, 1024)
write("\nAfter string create")
storage()
end
# Display current memory usages
procedure storage()
store := [] ; every put(store, &storage)
write("storage ", *store)
write("---------")
write("Static : ", store[1])
write("String : ", store[2])
write("Block : ", store[3])
end
Giving:
prompt$ unicon -s storage.icn -x
storage 3
---------
Static : 0
String : 0
Block : 34528
After string create
storage 3
---------
Static : 0
String : 131200
Block : 34808
See also
&subject¶
- Read-write
- Holds the current String Scanning subject, always a String.
When &subject
is explicitly assigned a new string value, &pos is
automatically set to 1.
#
# &subject, string scanning subject
#
procedure main()
str := &letters
str ? {
write(&subject, " at ", &pos)
move(10)
write("now at ", &pos)
&subject := &dateline
write("now at ", &pos)
}
end
Giving:
prompt$ unicon -s subject.icn -x
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz at 1
now at 11
now at 1
See also
&time¶
- Read-only
- Produces an Integer of the number of milliseconds (1/1000ths of a second) of CPU time that have elapsed since program execution began.
#
# &time sample
#
procedure main()
write("&time: ", &time)
end
Giving:
prompt$ unicon -s time.icn -x
&time: 4
This short program starts and completes in less than a tenth of a second under normal circumstances. Most runs of that example will show a relatively small number. Maybe as high as 100 if the system is extremely busy.
&trace¶
- Read-write
- Holds an Integer that determines the level of procedure depth for execution tracing.
Set to 0 (default) for no tracing, negative for infinite level tracing or to a
desired depth. Unicon examines the environment variable TRACE
on startup and
there is also a -t
compile time option to turn on tracing.
#
# &trace, procedure tracing to given level
# 0 means no tracing, negative is effectively infinite levels
# Unicon reads environment setting TRACE at startup
# There is also a -t compiler option, setting trace to ...
#
procedure main()
write("Initial &trace from -t: ", &trace)
write("Setting to 3 for this example")
&trace := 3
write("main level: ", &level)
second()
end
procedure second()
write("second level: ", &level)
third()
end
procedure third()
write("third level: ", &level)
fourth()
end
procedure fourth()
write("fourth level: ", &level)
end
Giving:
prompt$ unicon -s -t trace.icn -x
: main()
Initial &trace from -t: -2
Setting to 3 for this example
main level: 1
trace.icn : 20 | second()
second level: 2
trace.icn : 25 | | third()
third level: 3
trace.icn : 30 | | | fourth()
fourth level: 4
See also
&ucase¶
- Read-only
- Produces
#
# &ucase keyword, lower case letters Cset
#
procedure main()
write("Size of &ucase: ", *&ucase)
write(&ucase)
end
Giving:
prompt$ unicon -s ucase.icn -x
Size of &ucase: 26
ABCDEFGHIJKLMNOPQRSTUVWXYZ
&version¶
- Read-only
- Produces
#
# &version, Unicon version build information
#
procedure main()
write(&version)
end
Giving:
prompt$ unicon -s version.icn -x
Unicon Version 13.1. August 19, 2019
See also
&window¶
- Read-only
- Produces: Window
A variable containing a default window value. Most graphic functions will
default to using &window
when no initial window
argument is given.
#
# window.icn, demontrate the &window keyword
#
procedure main()
&window := open("default", "g", "size=90,60", "canvas=hidden")
# all subsequent graphic functions default to using &window
write("Colour depth (bits): ", WAttrib("depth"),
" on device ", WAttrib("display"),
" with window label ", image(WAttrib("windowlabel")))
Fg("vivid orange")
FillRectangle(30, 30, 30, 20)
Fg("medium cyan")
FillRectangle(35, 35, 15, 8)
# normally non graphic functions need to be told the window
Fg("black")
write(&window, "&window sample")
WSync()
WriteImage("../images/window.png")
close(&window)
end
Sample run:
prompt$ unicon -s window.icn -x
Colour depth (bits): 24 on device localhost:10.0 with window label "default"

&x¶
- Read-only
- Produces: Integer
Holds the horizontal mouse position. Set by calling Event.
#
# x.icn, demonstrate the &x mouse position keyword
#
link enqueue, evmux
procedure main()
window := open("mouse position", "g", "size=20,20", "canvas=hidden")
Enqueue(window, &lpress, 11, 14, "", 2)
Enqueue(window, &lrelease, 12, 15, "", 3)
w := Active()
write(image(w))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
close(window)
end
Sample run:
prompt$ unicon -s x.icn -x
window_1:1(mouse position)
event at mouse position (11,14)
event at mouse position (12,15)
&y¶
- Read-only
- Produces: Integer
Holds the mouse vertical position after Event is called.
#
# y.icn, demonstrate the &y mouse position keyword
#
link enqueue, evmux
procedure main()
window := open("mouse position", "g", "size=20,20", "canvas=hidden")
Enqueue(window, &lpress, 11, 14, "", 2)
Enqueue(window, &lrelease, 12, 15, "", 3)
w := Active()
write(image(w))
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
e := Event(w, 1)
write("event at mouse position (", &x, ",", &y, ")")
close(window)
end
Sample run:
prompt$ unicon -s y.icn -x
window_1:1(mouse position)
event at mouse position (11,14)
event at mouse position (12,15)
See also
Index | Previous: Functions | Next: Preprocessor